Creating a Simple Timer in Python and Using it with Programs in turtle
- Stephen Gruppetta
- Jul 5, 2022
- 4 min read
Updated: Feb 1, 2024
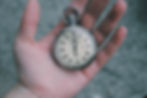
Creating a simple timer in Python for games or animations is not too difficult. In this article you will learn how to create and use a timer. You will:
Understand the difference between a Console and an Editor when writing Python code
Learn about the time module and the time.time() function
Find out how to start and stop a timer and make things happen when a certain amount of time has passed
Getting Started
You will use a module called time. Recall that a module is what we import in a program. You can think of this as fetching a book from the library that contains useful commands. In this case the book is called time and it contains commands to do with, you guessed it, time.
Coding in a Console
You will start using a Python Console. If you are using PyCharm or similar editors, find the Python Console option. In PyCharm it's at the bottom left of the window (if you cannot see the text Python Console in your version, click on the small square in the bottom left corner):
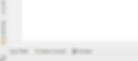
If you're using a web-based platform, such as a Trinket-based one, then you should be able to find a Console there too. On Trinket-based systems, including the Codetoday Unlimited learning platform, you can find it here:
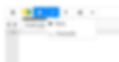
You know you're in a Console environment because each line starts with:
>>>
In a Console, each time you hit the Return (or Enter) key, that line of code is run (or executed). This is different to the usual setting (which we call writing a script) in which all the lines will run one after the other only when you press the Run button.
Using the time Module in Python
You can start by bringing the book called time from the Python library and running the function that is also called time from inside this book:
>>> import time
>>> time.time()
1599396434.8482718
The number you get will not be the same as the one above, but very similar. Can you guess what this number is?
This number tells us the time, but not in the way you're used to. It gives you the number of seconds that have passed since the 1st of January 1970. Strange? That's how computers keep track of time, using a date as a reference.
Why is this useful? Let's try the following:
>>> start = time.time()
>>> time.time() - start
6.587711811065674
You are now storing the time in a box called start when the first line runs. On the second line, Python checks the time again (when you write time.time()) but then takes away the time it checked when the first line was run. The number that you see is the number of seconds it took me between pressing Return after the first line and pressing Return after typing the second line. Try it out…
Showing a Timer in a turtle Animation
You can now go back to the usual place where you write your Python code in which you can write all of your code and press Run when you want to run the program.
Start by importing the two modules we need to put a timer in a turtle program, and create two turtles:
import time
import turtle
player = turtle.Turtle()
timer_text = turtle.Turtle()
The first turtle can be the player that moves in a game. In this example you will just make the player move continuously but when you use the timer in your future programs, your player turtle is likely to do more than just move!
You will use the second turtle to write text on the screen, in this case you will write the time that has passed.
Set the player so that it moves and turns continuously, but before it starts, we want to start the timer:
start = time.time()
while True:
player.forward(1)
player.left(1)
Next, you can write the time on the screen each time the player moves by adding:
timer_text.write(time.time() - start)
immediately after you make the player move (with the same indentation).
When you run this you'll notice something strange is happening. Each time the timer_text turtle writes the number of seconds elapsed on the screen, it writes the text on top of the previous text. Not good. Let's try adding
timer_text.clear()
before you write the text.
You now have a simple timer. If you are familiar with using tracer and update when using the turtle module, you can do so to make things smoother. You can also remove the numbers after the decimal point by changing the number to a whole number, which in Python is called an int (for integer). Here's the full code:
import time
import turtle
window = turtle.Screen()
window.tracer(0)
player = turtle.Turtle()
timer_text = turtle.Turtle()
start = time.time()
while True:
player.forward(1)
player.left(1)
timer_text.clear()
timer_text.write(int(time.time() - start))
window.update()
If you want to make the text look prettier, you can replace the line that writes the time on screen with:
timer_text.write(int(time.time() - start), font=("Courier", 30))
Remember that timer_text is a turtle like any other. So you can move it wherever you want on screen and change its colour, if you prefer.
Controlling How Long Things Happen in a Program
The final step in this short tutorial is to make the while loop run for only 5 seconds, let's say.
The while statement needs to be followed by something that Python understands as either True or False. You can therefore replace while True with:
while time.time() - start < 5:
Let's break this down. time.time() checks the current time whenever this line happens. You are then taking away the time at the start, just before the while loop starts. So time.time() - start, as you learnt above, will give the number of seconds that have passed since the player started moving.
You are then asking the computer to check whether this number is less than 5. So the program will understand time.time() - start < 5 as True when fewer than 5 seconds have elapsed but False once the time goes above 5 seconds.
Here is the final code:
import time
import turtle
window = turtle.Screen()
window.tracer(0)
player = turtle.Turtle()
timer_text = turtle.Turtle()
start = time.time()
while time.time() - start < 5:
player.forward(1)
player.left(1)
timer_text.clear()
timer_text.write(int(time.time() - start), font=("Courier", 30))
window.update()
turtle.done()
Your next task is to experiment a bit yourself with setting up and using timers. Have fun…
Enjoyed this and want to learn more Python for free? Check out our sister site The Python Coding Book, for in-depth guides on all things Python, or follow us on Twitter @codetoday_ and @s_gruppetta_ct