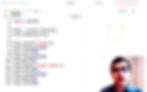
Never tried coding in Python before? Perhaps you have coded in Scratch, or maybe you have never done any coding before?
Coding using a language like Python can look very different to block coding like Scratch, but don't let that put you off. In this article you will learn how to write your first program using Python. Once you complete this lesson, you will then be able to continue exploring Python and write code to draw anything you can think of.
This lesson has two versions:
— The written article you are reading now that will guide you through the lesson
— A video that will also guide you through the same steps but in a different format
You can choose to use either the written text or the video, or even both if you prefer. Whatever works best for you.
Here is what you will learn:
— You will understand how Python works
— You will learn how to make drawings using turtle, a Python module
— You will learn about the basic grammar for coding in Python
To write and run your Python code you can go to this page: https://www.codetoday.co.uk/code I recommend you have both this page and the coding platform open at the same time and swap between them, or you can even have them open side-by-side.
Are you ready to start coding?
Python is a language. Like other languages that we speak, such as English, Python has words that have a certain meaning, and grammar which sets the rules on how to use these words. The punctuation is also very important in coding languages such as Python.
The first thing you need is to fetch a book from the library where Python keeps all its commands:
import turtle
This brings in a book called turtle which has lots of commands that you can use to draw in Python. It's a strange name for a book used to draw! You will see why it's called turtle soon!
To be able to draw, you will need to create a 'drawing turtle', this is a computer turtle (not a real one, of course!) that will move around the screen drawing lines and other shapes following your commands.
So time to create a turtle now:
fred = turtle.Turtle()
Does this line look a bit strange? Don't worry I'll explain what everything means. First, remember that this is not English or Maths, but Python. We are writing in a new language, so the rules are different to English or other languages.
First, I chose a name for the turtle. I called him fred, but you can choose another name is you prefer. There are some rules on naming the turtle, such as you cannot have spaces in the names. So, keep it simple for now! We also do not use capital letters at the start of the name.
The equals sign shows Python that you're going to tell it who fred is. To do this you then wrote turtle.Turtle(). The first turtle is the book with commands you have imported from the library on the first line. The second Turtle is the one that creates the drawing turtle. Notice how the second Turtle has a capital T and brackets at the end. These things are really important in Python, so we need to be very precise when we write code in Python.
Time to Draw
Let's ask fred to move and draw a line now. It would be nice if we could say:
"Hey Fred, could you please move forward a bit. Thank you."
That's English, but you need to speak to the computer in Python. Here's how we can translate the line above into Python:
fred.forward(100)
You have just asked fred to move forward by 100 steps, or pixels. Again, notice the punctuation marks we have used, and where. Try writing the three lines of code we have written so far in the coding platform (https://www.codetoday.co.uk/code) and press the play button.
Now, get some practice with getting fred to move around the screen, and get him to draw a square. You will see there is a new command in the code below, something else that turtles know how to do in Python:
fred.forward(100)
fred.left(90)
fred.forward(100)
fred.left(90)
fred.forward(100)
fred.left(90)
fred.forward(100)
fred.left(90)
By repeating the pattern of moving fred forward and turning him left four times, you got fred to draw a square for you!
Now see what happens if you add the following line immediately after you ask fred to move forward the first time:
fred.penup()
You can even draw a dot wherever you want in your code:
fred.dot(20)
Try putting this line in different places in your code, and try putting different numbers inside the brackets.
Some More Things Fred Can Do
I will now introduce two more commands and then it will be up to you to continue exploring.
Let's change the colour fred is using to draw. There is a command inside the turtle book called color. Notice how this is spelled in the American way, without a 'u'. There is also something else we need to do when using this command. Let's have a look:
fred.color('orange')
The name of the colour needs to go inside the 'quotation marks' as this is just the name of a colour, and not a special command that asks the computer to do something. Try changing fred's colour several times in your code, in different places.
And to finish off you can make fred look like a turtle by changing his shape:
fred.shape('turtle')
You can notice that again, we had to put the name of the shape inside quotation marks as turtle in this case refers to the name of the shape and not the book of commands be brought in on the first line. You can change fred's shape anywhere you wish in your program, as long as it's after the line where you tell Python who fred is (the one with the equals sign.)
Here is one version of this code, although I'm sure you can do better and draw something even more interesting than my version!
import turtle
fred = turtle.Turtle()
fred.shape('turtle')
fred.color('orange')
fred.forward(100)
fred.left(90)
fred.forward(100)
fred.dot(20)
fred.color('light green')
fred.left(90)
fred.forward(100)
fred.dot(20)
fred.left(90)
fred.forward(100)
fred.left(90)
fred.right(45)
fred.penup()
fred.forward(50)
Congratulations! You have just written your first computer program using Python. But don't stop there. Why not try to think of a shape and write code to draw it!
You can share your drawings with us in the comments below, if you wish.
Happy Coding in Python.